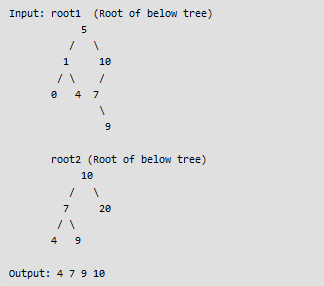
Binary Search Trees - C Program. Binary tree computations often involve traversals. Binary tree searching write search(v, k) Binary Search tree is a binary tree in. What are some practical applications of binary search. Binary Search Tree. All of the data-structures you cover in the CS program is very useful to have a good. Binary search trees. What are some practical applications of binary search trees. All of the data-structures you cover in the CS program is very useful to.
In a binary tree, every node can have maximum of two children but there is no order of nodes based on their values. In binary tree, the elements are arranged as they arrive to the tree, from top to bottom and left to right. A binary tree has the following time complexities.
• Search Operation - O(n) • Insertion Operation - O(1) • Deletion Operation - O(n) To enhance the performance of binary tree, we use special type of binary tree known as Binary Search Tree. Binary search tree mainly focus on the search operation in binary tree. Binary search tree can be defined as follows. Binary Search Tree is a binary tree in which every node contains only smaller values in its left subtree and only larger values in its right subtree. In a binary search tree, all the nodes in left subtree of any node contains smaller values and all the nodes in right subtree of that contains larger values as shown in following figure. Example The following tree is a Binary Search Tree.
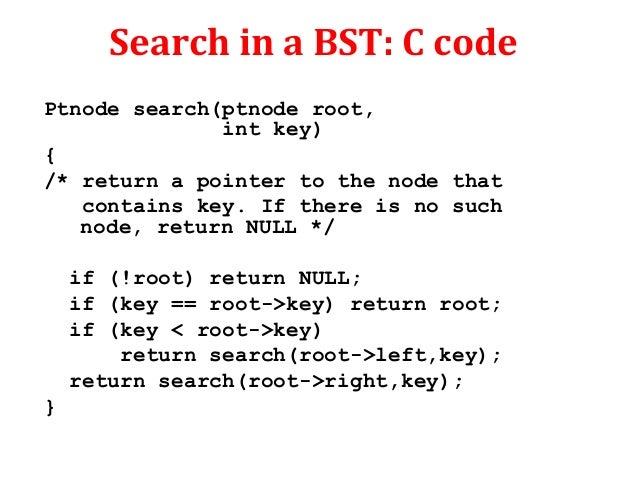
In this tree, left subtree of every node contains nodes with smaller values and right subtree of every node contains larger values. Every Binary Search Tree is a binary tree but all the Binary Trees need not to be binary search trees.
Operations on a Binary Search Tree The following operations are performed on a binary earch tree. • Search • Insertion • Deletion Search Operation in BST In a binary search tree, the search operation is performed with O(log n) time complexity. The search operation is performed as follows. • Step 1: Read the search element from the user • Step 2: Compare, the search element with the value of root node in the tree.
• Step 3: If both are matching, then display 'Given node found!!!' And terminate the function • Step 4: If both are not matching, then check whether search element is smaller or larger than that node value. • Step 5: If search element is smaller, then continue the search process in left subtree. • Step 6: If search element is larger, then continue the search process in right subtree. • Step 7: Repeat the same until we found exact element or we completed with a leaf node • Step 8: If we reach to the node with search value, then display 'Element is found' and terminate the function. • Step 9: If we reach to a leaf node and it is also not matching, then display 'Element not found' and terminate the function.
Insertion Operation in BST In a binary search tree, the insertion operation is performed with O(log n) time complexity. In binary search tree, new node is always inserted as a leaf node. The insertion operation is performed as follows. • Step 1: Create a newNode with given value and set its left and right to NULL. Metin2 Yang Hack Exe V1 81 Games. • Step 2: Check whether tree is Empty.
• Step 3: If the tree is Empty, then set set root to newNode. • Step 4: If the tree is Not Empty, then check whether value of newNode is smaller or larger than the node (here it is root node). • Step 5: If newNode is smaller than or equal to the node, then move to its left child. If newNode is larger than the node, then move to its right child.
• Step 6: Repeat the above step until we reach to a leaf node (e.i., reach to NULL). • Step 7: After reaching a leaf node, then isert the newNode as left child if newNode is smaller or equal to that leaf else insert it as right child. Deletion Operation in BST In a binary search tree, the deletion operation is performed with O(log n) time complexity. Deleting a node from Binary search tree has follwing three cases. • Case 1: Deleting a Leaf node (A node with no children) • Case 2: Deleting a node with one child • Case 3: Deleting a node with two children Case 1: Deleting a leaf node We use the following steps to delete a leaf node from BST.